12 Oct 2019
I have a fascination with Lisp / lisp like languages. This started way back when I joined the Emacs religion, part of the initiatiion is to configure your IDE via macros! Much later I tried creating (and failed to maintain) a emacs package that would interact with Bugzilla.
Another experiment was to use a Sawfish Window Manager , which is highly configurable via a lisp like programming language rep. This was the early days of Linux desktop, with a plethora of window managers.
As of today I have been programming with Clojure for few months now. A lot has been written about pro’s and con’s of Clojure. However in this post I want to share with you why Lisp from a perl programmer’s perspective (perl is the one language I revert to for any proof-of-concept project) - Why Lisp macros are cool, a Perl perspective
I’ll leave this quote from the link above, which is a sufficient reason for me to stick with lisp:
Source code generation is unreliable and inadvisable in every language
except Lisp.
08 Feb 2018
I recently stumbled upon Elm, a functional reactive programming. I literally spent evenings and nights for
the next 2 weeks pouring over tutorials and videos learning Elm.
I found these resources really helpful:
I ported over a search widget to Elm (from KnockoutJS) and that helped cement much of what I learned. The backend is written in Mojolicious.
I even published my first CPAN module , Mojolicious::Plugin::AssetPack::Pipe::ElmLang -
a pipeline to compile Elm source files into Javascript!
The journey so far has been smooth sailing and Im really enjoying learning
05 Jan 2018
Objective-C provides a very concise way of accessing values of any object (that supports NSKeyValueCoding protocol).
Here’s my attempt at implementing a simple KVC type accessor for PHP arrays.
/**
* value_for_keypath
*
* returns value at the given key path in an multi-dimensional array (map)
*
* @param $array
* @param $path string , each path element is separted by a dot (.)
*/
function value_for_keypath($input, $path)
{
$keys = explode( ".", $path );
$tmp_val = $input;
foreach ($keys as $key) {
if (isset( $tmp_val[$key] )) {
$tmp_val = $tmp_val[$key];
} else {
return null;
}
}
return $tmp_val;
}
Eg:
php > $a = array('a' => array('b' => array('c' => 'test')));
php > echo value_for_keypath($a , "a.b.c");
test
Note: PHP is a very forgiving language, one can access non-existent keys in a deeply nested multi-dimensional array and at runtime only a notice is generated, eg:
php > $a = array('a' => array('b' => array('c' => 'test')));
php > echo $a['a']['b']['d'];
PHP Notice: Undefined index: d in php shell code on line 1
PHP Stack trace:
PHP 1. {main}() php shell code:0
02 Oct 2017
Simplicity is prerequisite for reliability.
– Edsger W. Dijkstra
One of the common problem with web-based application is how to handle database migration. Often times the answer to this is to use the migration tool that comes with the ORM the system relies on.
In the past, as the system grew to use multiple layers each with its own runtime / language / ORM , I was forced to maintain db migration in one of the layer. Thus I came to realize that an ORM agnostic db migration tool would be the ideal approach.
One of the simplest db migration tool I have encountered is shmig - A database migration tool written in BASH consisting of just one file! Shmig even supports creating test data (which is another gnarly issue worth a blog post).
If you are looking for a db migration tool I reccomend you take a look at shmig.
15 Aug 2017
Continuing my blog theme on repl’s whats more appropriate than a blog about shells! Thus far I have been using zsh with oh my zsh. In itself zsh
is good however it had gotten noticeably slow. I attribute this to the git plugin.
Enter Friendly Interactive SHell! In simple words - fish:zsh::zsh:sh
.
I no longer need to muck around config files, fish_config
is a shining example of how CLI applications should handle configuration. Here’s a screenshot of fish_config
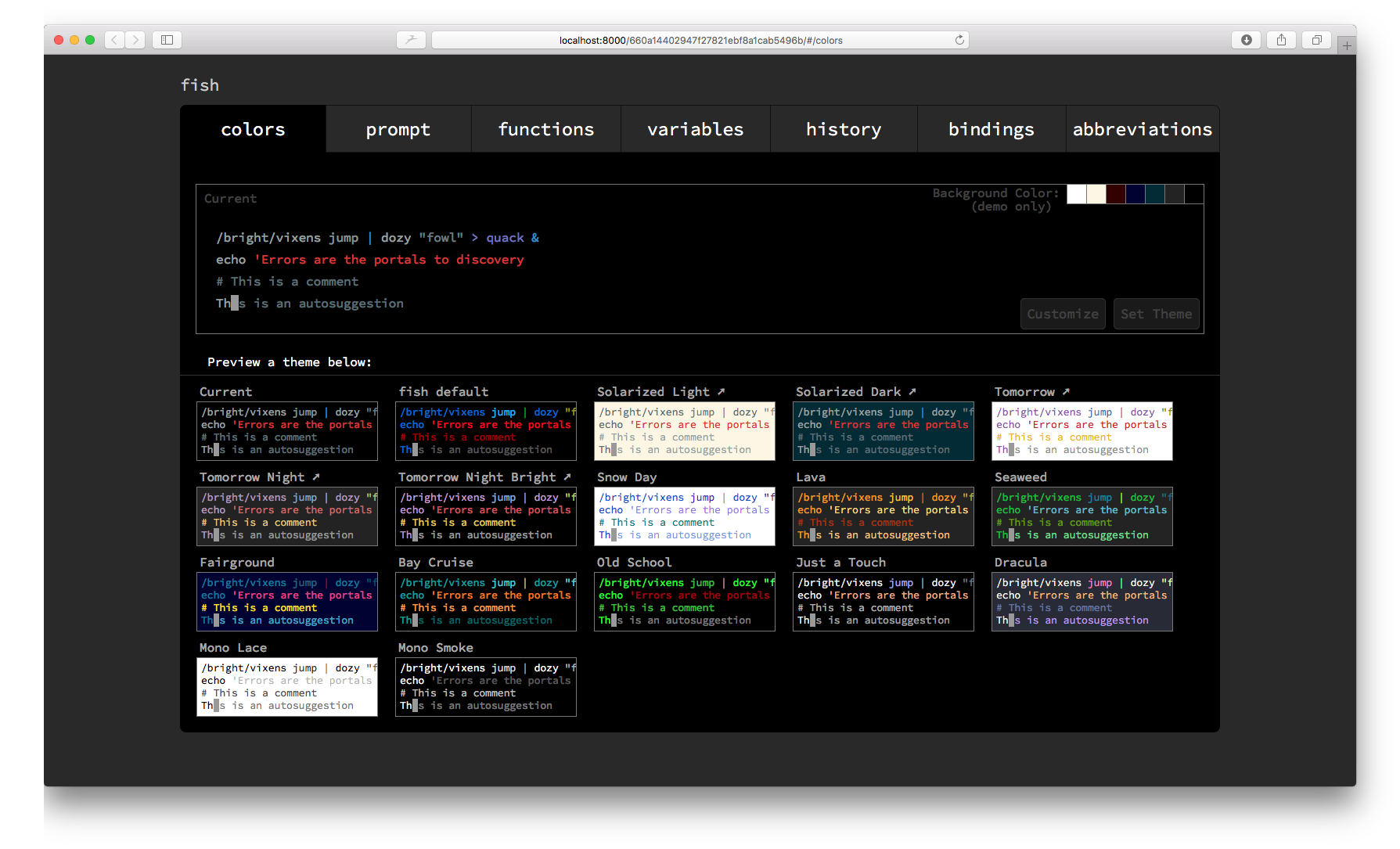
Combine this with fisherman you have a very responsive and a very user friendly shell.